StoryDevs Events: Timezone Hell
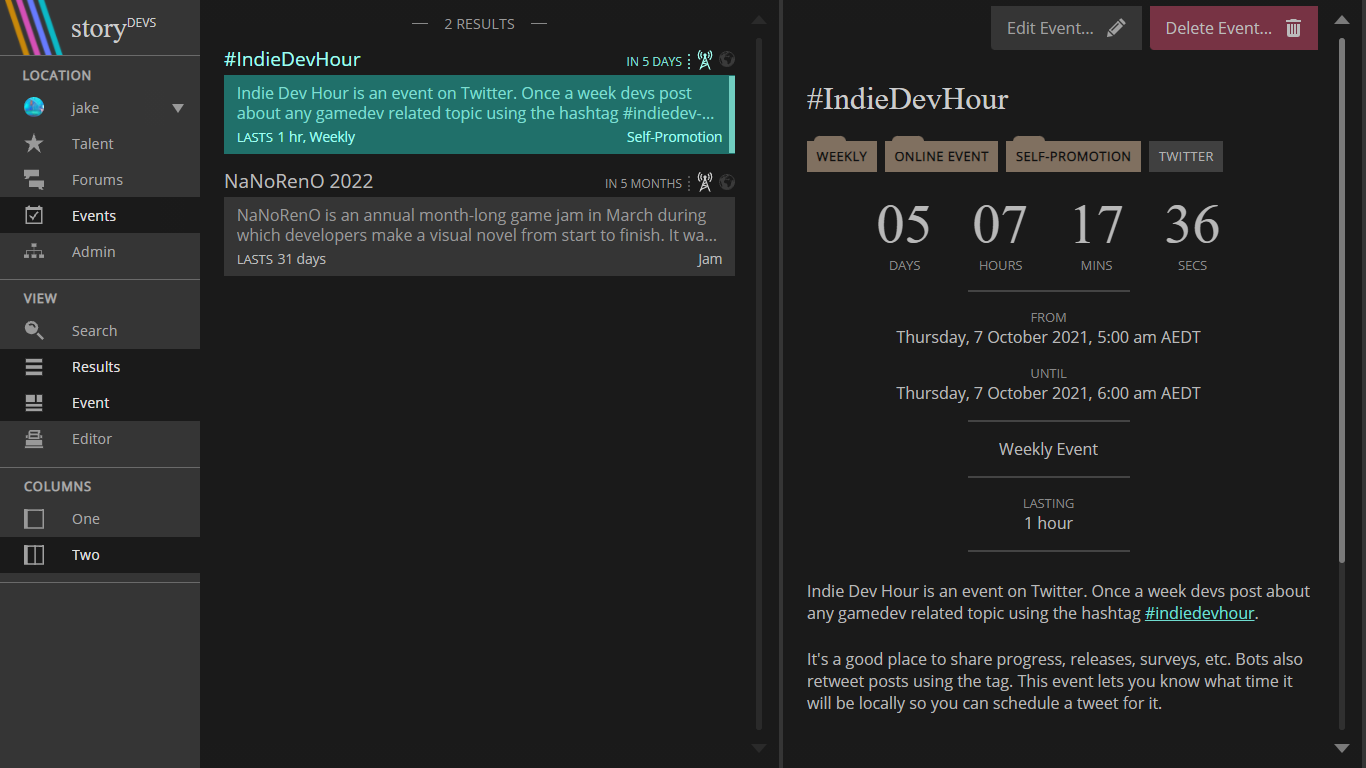
Recently I implemented an event database on StoryDevs. It allows users to create an event whose date and time is automatically translated into the viewer's local timezone. It features quite sophisticated creation and search tools:
- Events can have a duration (6pm - 9pm) or be instantaneous (for example the launch of a product at 1pm).
- Events can be occur at a singular moment in time or in local time. New Year's would be a local time event as it happens at different points in time around the world but the same local time for everyone.
- Events can be one-off or they can be recurring. The latter automatically reset their countdown and update their displayed date without the creator having to edit things.
- Searches can be strict and only return results within the specified time range or they can be permissive and show events that at least overlap the range selected.
- Searches allow you to specify days of the week that interest you and times therein. For example Mondays through Fridays, 6pm - 9pm.
- Searches can auto-detect your timezone so that you don't have to even know what it is but there is a manual override if you happen to be using a proxy.
Today I'm going to explain the basics of how this was achieved.
How Dates Are Stored
StoryDevs uses a SQL database, Postgres. All dates are stored within it as Unix timestamps along with the timezone in a separate field. This might seem an odd choice since Postgres supports date types and allows you to do a number of useful things with them.
CREATE TABLE IF NOT EXISTS event (
-- Elide other fields...
timezone text NOT NULL,
start bigint NOT NULL,
finish bigint,
weekly bool
)
However I think it's easier to use a timestamp than a date due to the things we're doing in the below examples, particularly when it comes to calculating the next occurrence of recurring events. Perhaps I'm mistaken about that.
Instant Vs. Not
We have events that last some period of time: an hour, a day, a month such as conferences, game jams, etc. Then we have events that have no duration at all like product launches. Based on the table definition above this means finish
may or may not be NULL
— we always need to check:
SELECT
*
FROM
event
WHERE
start >= $1 AND
(
(finish IS NOT NULL AND finish < $2) OR
(finish IS NULL AND start < $2)
)
This is a simple check to gather all the events that fall completely within the client's beginning and end range (represented by $1
and $2
respectively). It captures both events with a duration and instant events. This is not a tricky query but it becomes complex as we begin to add to the number of simultaneous considerations.
Overlapping vs. Not
Next up consider that our search allows strict queries (like the example above) as well as more permissive queries that can tolerate partial overlaps. If the client searches for events between 6pm and 9pm, toggling on the overlap setting shows events that at least begin or end within that range:
SELECT
*
FROM
event
WHERE
(start >= $1 AND start < $2) OR
(finish IS NOT NULL AND
finish >= $1 AND finish < $2)
Again, quite simple. But we need to write both because we don't know which it is until the client submits the search. So these two previous examples exist in two different branches of an if
statement.
Local Vs. Not
Here's where the timezone column comes in. We need to show both local and non-local time events in our results. But we check whether they're in range differently. The first OR paranthetical below shows us subtracting $3
(representing the client's timezone offset) from the dates to ensure they're the right local time.
SELECT
*
FROM
event
WHERE
(
timezone = 'local' AND
(start - $3) >= $1 AND
(
(finish IS NOT NULL AND (finish - $3) < $2) OR
(finish IS NULL AND (start - $3) < $2)
)
)
OR
(
timezone != 'local' AND
start >= $1 AND
(
(finish IS NOT NULL AND finish < $2) OR
(finish IS NULL AND start < $2)
)
)
Now we're starting to see how multi-dimensional this is getting. The above query is for strict ranges. A mirror version needs to exist for the overlap-permitting version too, which I'll leave to your imagination.
Recurring Vs. Not
Lastly, we need to consider that events can automatically recur week after week. Solving this resulted in several false eureka moments for me! I kept thinking I'd fixed it only to realise I hadn't.
Originally I addressed this by waiting for a user to perform a search. Before searching we check all recurring events have finish dates (or start dates if they have no finish date...) after the present moment. If they'd "finished" we updated the date to the next occurrence.
This doesn't work. And that's because a singular local event will update at different moments in time across the world. You can't update it without making it incorrect for someone else.
Instead, I preserve the original the original date and do the calculation during the query. This, it seemed from my cursory research (I may be wrong!), was better suited to a Unix timestamp. It's possible to convert a Postgres date to a timestamp (I do it below) but honestly there's enough shit going on in this query without EXTRACT(bla bla bla)
everywhere. I think this is easier to read.
WITH v (now, wk) AS (
VALUES(
(SELECT EXTRACT(EPOCH FROM now()))::BIGINT,
60 * 60 * 24 * 7
)
)
SELECT
*
FROM
event
WHERE
weekly AND
now + (wk - ((now - start) % wk)) >= $1 AND
(
(finish IS NOT NULL AND
now > finish AND
now + (wk - ((now - finish) % wk)) < $2) OR
(finish IS NULL AND
now > start AND
now + (wk - ((now - start) % wk)) < $2)
)
I use the WITH
clause to effectively create two variables: now
and wk
(meaning week). Both now
and wk
are measured in seconds like our start and finish dates.
Let's just look at one subcomponent of the query:
now + (wk - ((now - start) % wk)) >= $1
If we start from the inner paratheses this isn't so bad. First we subtract the start date from now. The difference might be hours, a day, 3.7 weeks, etc. Then we use the modulo operator to get the remainder of that difference divided by a week. So if the difference were 3.7 weeks we'd get whatever 0.7 weeks is in seconds as our result.
So now we have how much time in the week has passed since the last occurrence minus any whole weeks. Next we invert that by subtracting this fraction of a week from a whole week. The result is how many seconds until the next occurrence minus any whole weeks. Then we simply add this onto now
to get an absolute date.
Non-SQL Code
Thus far I've simplified the queries to give you an idea of what's happening which has given me a chance to explain why each component is the way it is. Soon we'll get to how I generate the full query. It's a little lengthy though and requires some preamble.
Building queries on the server side involves a lot of condtionals, string concatenation of SQL code, and some string interpolation. However it's very important not to naively use client input without escaping it otherwise we'll end up with a SQL injection vulnerability. This is why we've been using prepared statements with $n
so far.
In Postgres you must number your escaped values. This is fine for handwritten queries with a couple of values you wish to insert. But when it comes to 20 or 30 it gets hard to keep track of. So I have a simple type I created to keep count and produce the placeholder strings:
type argCount int
func (ac *argCount) Next() string {
*ac++
return "$" + strconv.Itoa(int(*ac))
}
When you see arg.Next()
below know that it's yielding an incremented string value like $5
, $23
, etc etc.
As we build this query we're not just concerned with date ranges. There's categories, settings, and so on that are also being conditionally added. So at the top scope of the generating function we have two slices, where
and args
. (If you're not familiar with Go a slice is similar to a Python list or a JavaScript array but with type safety.)
where = append(where, "category = " + arg.Next())
args = append(args, category)
Once we've finished adding conditions we'll join the where statements together and concatenate it to the rest of the query. Then it and the args slice will be supplied to a database driver function which escapes our arguments and performs the query for us.
q := `
SELECT
*
FROM
event`
/*
Loop through search filter and
append conditions...
*/
q += " WHERE " + strings.Join(where, " AND ")
var results []event
err := db.Select(&results, q, args...)
if err != nil {
return err
}
return results
Combining Them All
Now we're ready to look at (almost) everything combined. Below is how I generate the overlapping branch of the query. As mentioned above there is a parallel strict branch that I won't show here since it's very similar to what's below.
qMaker := func(opening string, offset int) string {
off := ""
if offset > 0 {
off = " - " + strconv.Itoa(offset)
}
s := "
/*
Events set to local timezone have the
user's timezone offset subtracted from
event start/finish.
*/
%s AND
(
start %s >= %s AND
start %s < %s
)
OR
(
finish IS NOT NULL AND
(
(finish %s >= %s AND
finish %s < %s) OR
(start %s < %s AND
finish %s >= %s)
)
)
OR
(
-- For recurring events.
weekly AND
(
(finish IS NOT NULL AND now > finish %s) OR
(finish IS NULL AND now > start %s)
)
AND
(
(now + (wk - ((now - (start %s)) %% wk)) >= %s AND
now + (wk - ((now - (start %s)) %% wk)) < %s) OR
(finish IS NOT NULL AND
now + (wk - ((now - (finish %s)) %% wk)) >= %s AND
now + (wk - ((now - (finish %s)) %% wk)) < %s)
)
)
"
return fmt.Sprintf("("+s+")",
opening,
off, arg.Next(), off, arg.Next(),
off, arg.Next(), off, arg.Next(),
off, arg.Next(), off, arg.Next(),
off, off,
off, arg.Next(), off, arg.Next(),
off, arg.Next(), off, arg.Next())
}
var ss []string
ss = append(ss, qMaker(`timezone = 'local'`, offset))
ss = append(ss, qMaker(`timezone != 'local'`, 0))
where = append(where, "("+strings.Join(ss, " OR ")+")")
args = append(args,
s, f, s, f,
s, f, s, f,
s, f, s, f,
s, f, s, f,
s, f, s, f)
First I define a nested function which is done for readability purposes. It's easier to understand how the query is being built if it procedes A, B, C rather than having to jump around the code. (I called it qMaker as in "query maker" - honestly, I didn't know what the fuck to call it.)
This function allows us to create local and non-local versions of the query. For local versions we pass in the client's current timezone offset and we create a little string for interpolation that ends up being " - 10"
, " - -7"
, etc if a non-zero offset is supplied or an empty string otherwise.
The query is basically a combination of the aforementioned so I won't really go over it. We're checking if the event period as entered in the database overlaps the client's desired range or, if not, if the next calculated occurence of it overlaps. All while being mindful that finish
may be NULL
.
The only part that's new is this:
weekly AND
(
(finish IS NOT NULL AND now > finish %s) OR
(finish IS NULL AND now > start %s)
)
We're checking that any recurring event's original finish date is in the past (keeping in mind that the start could also be the finish date). This prevents recurring events being backwards projected into the past where they never actually occurred.
Then we format the string passing in a whole bunch of values to be interpolated, mostly the client offset and the placeholder $n
.
We call the function twice and append the resulting string to a temporary slice ss
which is then joined together on either side of an OR
operator. This results in something like:
(
(
timezone = 'local' AND
-- The rest of the local conditions...
)
OR
(
timezone != 'local' AND
-- The rest of the non-local conditions...
)
)
Which saves us the time and space of writing basically the same thing twice. This in turn is appended to where
and the ranges supplied by the client are appended to args
. Since qMaker
is called twice we need to supply double the amount of arg.Next()
calls shown.
Honestly, the way this works is wasteful. Postgres has numbered placeholders $1
, $2
, and on which allow repeatedly referring to one argument. The s
and f
arguments really only need to be supplied once each. Perhaps I'll alter it in the future to do this.
Conclusion
Well that's all I can be bothered writing for now. I hope this has been clear enough to give an idea of how things work. Perhaps in a future post I can describe how searches for specific days of the week work, though it's not too dissimilar from calculating recurring events.
Thanks for reading.